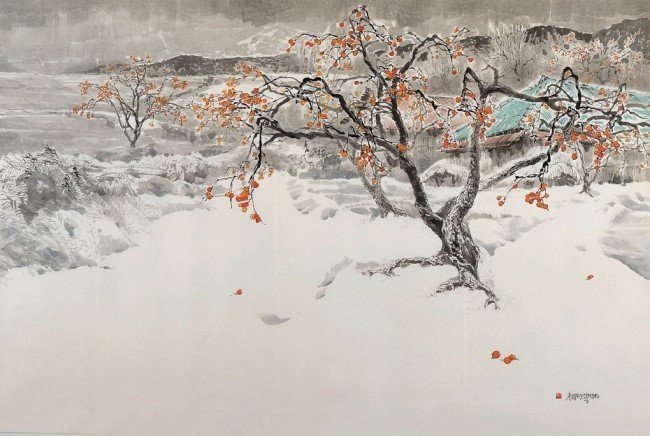
redux toolkit createAsyncThunk
export const fetchRoomsByPlace = createAsyncThunk(
'home/fetchRoomsByPlace',
async (params : any, { getState, requestId } : any) => {
const { currentRequestId = '', loading } = getState().homeReducer;
if (!_.isEqual(loading, LOADING_TYPE_ENUM.PENDING || !_.isEqual(requestId, currentRequestId))) {
return null;
}
const { place = DEFAULT_PLACE } = params;
const response = await axios.get(`${API_URL}?place=${place}`);
return response.data;
},
);
const homeSlice = createSlice({
name: 'homeReducer',
initialState,
reducers: {
setDataFromIndex(state, action) {
state.loading = action?.payload?.loading ?? false;
},
},
extraReducers: {
[fetchRoomsByPlace.pending]: (state, action) => {
if (_.isEqual(state.loading, LOADING_TYPE_ENUM.IDLE)) {
state.loading = LOADING_TYPE_ENUM.PENDING;
state.currentRequestId = action.meta.requestId;
}
},
[fetchRoomsByPlace.fulfilled]: (state, action) => {
if (_.isEqual(state.loading, LOADING_TYPE_ENUM.PENDING)
&& _.isEqual(state.currentRequestId, action.meta.requestId)) {
state.loading = LOADING_TYPE_ENUM.IDLE;
state.rooms = action.payload?.rooms ?? [];
state.currentRequestId = undefined;
}
},
[fetchRoomsByPlace.rejected]: (state, action) => {
if (_.isEqual(state.loading, LOADING_TYPE_ENUM.PENDING
&& _.isEqual(state.currentRequestId, action.meta.requestId))) {
state.loading = LOADING_TYPE_ENUM.IDLE;
state.error = action.error;
state.currentRequestId = undefined;
}
},
},
});
const { rooms, loading } = useSelector((state: rootState) => state.homeReducer);
const dispatch = useDispatch();
useEffect(() => {
dispatch(fetchRoomsByPlace({ place: DEFAULT_PLACE }));
}, []);
